This is an intro to JavaScript web applications. It's the first of two posts that comprise a primer on this topic (with emphasis on SPAs).
JavaScript Web Applications
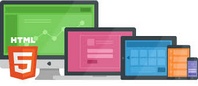
Web developers have been using client-side JavaScript for years, but it was always a bit player, adding some interactivity to a page and maybe doing some background work like data validation but in the end it was always the server running the show. However, recently JavaScript has begun to take a more prominent role, more and more found driving large browser-based applications similar to desktop applications. This post considers what JavaScript web applications are and when they're a good architectural choice for an application.
The term "JavaScript web applications" is a bit nebulous so let's start by defining what we're talking about. First, a JavaScript web application is not just a page that makes use of JavaScript to create slick effects or to perform forms validation. JavaScript web applications are about moving processing and data from the web server to the client. A primary goal is to create web applications with functionality as close to desktop applications as possible, relying little (or not at all) on a server to generate views. Emphasis is on running code and manipulating data on the client for improved performance and interactivity, resulting in a better UI and thus a better user experience.
![]() |
To keep things simple I'll describe the client interacting with "the server" here, but these days your application may interact with many servers or cloud services. |
Obviously a JavaScript web application is driven by JavaScript, which means it's client-side and browser-based (we're not talking Node.js here). In this architecture the server's role is mostly supportive, that of data store and compute server. Here's a schematic from a MSDN post that reflects this (note how little work is done on the server side).
In this architecture the only "normal" browser/server interaction is often the initial page load triggered by your application's URL. This page load initializes the application, loading the application core. However, once your application is up and running it doesn't drive things through URLs. Instead, it makes "background" calls to the server (calls that don't trigger a page load), and these calls are made only as the application needs more data or other resources. Navigation to (and generation of) new pages or parts of pages is primarily done on the client using JavaScript and either local data or data pulled on demand from the server via asynchronous fetches (XHR/Ajax). Generated content is often tailored to the data it's displaying through the use of templates which contain HTML and possibly some JavaScript. These templates are combined with data at runtime to create valid markup that is then injected into the DOM (part 2 of this primer covers all of this in more detail)
Compare this to the traditional model where the client relies on the server for its content, a constant request-and-response interaction where the server generates content based on the client's URL requests and the client mostly just loads and renders the server's response (with of course some embedded js to provide a little interactivity).
One big difference between these two approaches is user experience — the client-driven approach can provide a smoother user experience similar to a desktop application while the constant page reloads of the server-driven approach disrupt the user experience (and provide poorer performance).
JavaScript web apps that have only a single page load are called Single Page Applications, or SPAs. Here's a definition of SPAs from Addy Osmani (who has many great posts on this topic — see References section below).
"What’s A Single-Page Application (SPA)?
SPAs are web applications or websites which persistently run in the same page without requiring a reload for further navigation. All of the code needed for your initial load in these applications is driven by either local data or data retrieved from a web server on demand, such as any additional data required from your app that might be driven by user actions.
The basic idea behind an SPA is that regardless of what interactions your users might have with the application, the page doesn’t get reloaded or have it’s control handled by another page outside of the current one."
From Building Single Page Applications With jQuery’s Best Friends
![]() |
In addition to Single Page Application (SPA) you'll also see the term Single Page Interface (SPI). |
It's important to note that there's no sharp boundary between server-driven web applications and client-driven web applications. Think of it as a continuum — on one end are applications highly reliant on the server and with minimal client interactivity, on the other end you have client-driven SPAs. However, along this continuum you can also have "part-time SPAs" which may have some full page reloads but these might be few and far between, with pages persisting for significant periods and providing a high level of client-side processing. Look at this bit from MSDN's project Silk Chapter 1 (see Resources section below for more on this MSDN project):
"There is a spectrum of web applications being built today that can be grouped into four application types. These types of web applications are categorized by their full-page reload behavior and the amount of client-side interactivity they provide. Each application type provides a richer experience than the one listed before it.
- Static sites. These consist of static HTML pages, CSS, and images. They are static in that as each page is navigated to, the browser performs a full-page reload and there is no interaction with portions of the page. In addition, the page does not change no matter who requests it or when.
- Server rendered. In this model, the server dynamically assembles the pages from one or more source files and can incorporate data from another source during the rendering. The client-side script in these applications might perform some data validation, simple hover effects, or Ajax calls. As each page is navigated to, the browser performs a full-page reload. ASP.NET applications that don't make heavy use of client-side JavaScript are examples of server-rendered web applications.
- Hybrid design. This model is similar to the server-rendered web application, except that it relies heavily on client-side JavaScript to deliver an engaging experience. This type of application has islands of interactivity within the site that do not require full-page reloads to change the UI as well as some pages that do require a full-page reload. Mileage Stats is an example of a hybrid design.
- Single-page interface. In this model, a full-page load happens only once. From that point on, all page changes and data loading is performed without a full-page reload. Hotmail, Office Live, and Twitter are examples of single-page-interface web applications."
from MSDN's Project Silk: "Chapter 1: Introduction"
While I think both of those last two categories qualify as JavaScript web applications this primer will focus on SPAs, not hybrids, and I'll use the terms "JavaScript web app" and "SPA" interchangeably. Note that the JavaScript Data Explorer demo app that accompanies these posts is a SPA.
So, what makes up a JavaScript web application? Here's a summary of the primary features:
- minimal page loads (for SPAs, a single page load)
- emphasis on client-side execution with little or no reliance on server for generating views. Navigation and application state is also managed/maintained on the client
- data copied to the client where it can be manipulated (e.g., filters, sorts) and used to generate views without use of server processes (this isn't a hard and fast rule – when dealing with large datasets some server-side processing may be preferable). Data is also often cached and even prefetched.
- server requests are asynchronous, usually as Ajax calls with results handled by success/failure callbacks. As a result a js web app is generally non-blocking, i.e., the page does not "freeze up" while a server request is pending.
- rich UI with emphasis on user experience including fluid state changes (often with transition effects). Components are usually stateful and incorporate lifecycle events such as create, init, destroy.
While not required, SPAs also have some frequently found traits, such as employing an MV* lib/framework, loose coupling via an event bus (e.g. PubSub), use of the JSON data format.
Much of the above has been used in web development for awhile but only recently has all of this been aggregated into highly functional web applications. This has largely been driven by recently introduced standards (e.g., HTML5, CSS3) , better JavaScript performance (e.g., Google's V8), and especially by new developer libraries and frameworks (e.g., Backbone, Ember, Sencha).
As you might expect, all of this can be a heavy lift. Maintaining data on the client, keeping local data in sync with your backend master copy, dealing with more functional (and complicated) UI components, managing your app's state and navigation, and learning a constantly changing/expanding set of libs and tools has a cost. But if your goal is to create highly functional applications that run on many platforms these costs can be worth the benefits. These benefits are the focus of the next section.
Why JavaScript web applications?
When developing an application you may have a choice of architecture — traditional server-driven web application, or client-side web application (SPA), or even a desktop or a native mobile application. So, what are some pros and cons of JavaScript web applications, and how do they compare to these other approaches?
As we've seen, JavaScript web applications have advantages over traditional server-driven web apps:
- UI more like desktop applications, without the constant disruption of page reloads
- performance can be better because the application can respond without making a server request
- client can talk directly to cloud services (though cross-domain issues may require use of JSONP or CORS)
- reduced web server load
On the other hand, SPAs don't compare so well to server-based web applications in other respects:
- The user may have JavaScript disabled. Of course this is also a problem for server-driven applications whose pages include some JavaScript but it's especially problematic for SPAs which are reliant on JavaScript to drive the application. You can try to deal with this through progressive enhancement, but without JavaScript you'll usually end up with a fundamentally different application
- search engine indexing is harder. Crawlers won't "see" all of your content unless you do extra work to make things visible. If SEO is important to you then this can be a major drawback (tho HTML5 PushState helps with this)
- development of SPAs is generally more complex/costly than server-driven applications (though of course in the end you get more for your money, e.g. better UI/UX)
When comparing server-driven to client-driven web applications the nature of the application is key — if you're primarily surfacing static content like documents and images then server-driven is probably the best bang for the buck, but if your application is highly interactive, especially if it is letting the user explore data (what-if analysis, interactive graphs, etc.) then you should consider a SPA.
The comparison of SPAs to desktop and mobile apps is more complicated, but SPAs do have several advantages, many deriving from being browser-based:
- your execution context is always available, since browsers are everywhere
- there is the advantage of zero install — users will always have the latest version of your app, not a stale locally installed version that needs to be updated (so no need to support old versions, either)
- potential for cross-platform application (unlike native mobile apps, for example, which are platform-specific). This is especially true if you are just targeting HTML5/mobile. more on this below...
- bypass native app stores – no limit on content, no censorship
It's worth highlighting the cross-platform potential of SPAs, especially in the heterogeneous world of mobile. For example, to deliver an application's functionality via a native app on both iOS and Android you'll need 2 development teams and end up with 2 codebases. Obviously you want to avoid this if you can — bad enough to double your development work, but these costs compound when you add ongoing maintenance (applying bug fixes, keeping the versions in sync as you release new features, etc.). The SPA approach can maximize code reuse, with minimal target-specific code (variances are mainly related to the presentation layer, which needs to adjust to the display it's running on and maybe styled to the target's UI conventions). Still, there are limitations to what you can do, and once again many of these are related to being browser-based (e.g., you are constrained by the browser's security sandbox).
![]() |
Regarding cross-platform applications, there are other options like Titanium Appcelerator, PhoneGap and AIR. I cover these very briefly in part 2 of this primer. |
So, how do SPAs stack up against native desktop and mobile applications? There definitely are some drawbacks:
- while browsers are everywhere there are differences between them. This can be a signficant challenge when you need to support older desktop browsers. Note that mobile browsers, while better, still have signficant differences, less with JavaScript than with styling and layout.
- a SPA's initial load will take longer than a native app since your code needs to load from a remote server (though caching can help); in general performance isn't going to be as fast as a native app's
- If the device has no network connection then your application won't load. And creating an offline web app is still hard
- a browser-based SPA will be playing in that browser security sandbox, with restricted access to file system and OS APIs
- related to above, browser-based apps don't have the same device access as a native app — for example you have geolocation and accelerometer but no camera access yet (though there are hybrid workarounds like PhoneGap)
- not fully native in look and feel. For appearance you can come close with clever CSS, but without using native widgets you won't have full native functionality (e.g., Android text input has built in spellcheck, your web control won't act exactly the same).
- JavaScript web apps aren't in a store (e.g., iTunes) so may be harder to find. This also makes them harder to monetize.
- one-touch access to a web app is hard on some mobile
On Javascript
JavaScript has long had a bad reputation with many programmers, and some would say that JavaScript itself is a weakness of SPAs. However, while JavaScript did have problems in the past, largely with performance and with inconsistencies in implementations (as often is the case, Microsoft moved to a different drummer than other browser vendors) many of the complaints against JavaScript were really problems arising from browsers and the DOM. In any case, this situation is much improved, and JavaScript performance should be adequate for most applications (but if you're targeting mobile you'll need to pay close attention to optimizing your code because of mobile's limited processing power and memory; on mobile performance is still an issue).
Another JavaScript topic worth a mention is the handling of browsers where JavaScript has been disabled. In general you'd like your application to always work in this case, and you should use progressive enhancement where it's feasible. But for some web applications this might not be necessary or worth the effort or even possible. Here's a bit from David Flanagan's great "JavaScript: The Definitive Guide" on this topic:
"JavaScript is more central to web applications than it is to web documents, of course. JavaScript enhances web documents, but a well-designed document will continue to work with JavaScript disabled. Web applications are, by definition, JavaScript programs that use the OS-type services provided by the web browser, and they would not be expected to work with JavaScript disabled."
From section 13.1.2 of "JavaScript: The Definitive Guide" 6th edition
Conclusion
Over the next few years as older browsers are replaced and as new APIs become available to mobile browsers we're likely to see many more JavaScript web applications. Of course, there's a place for all types of applications, from server-based web applications to native apps on mobile devices to, yes, even traditional desktop applications. JavaScript web applications are simply one more option, with pros and cons like any other application architecture. Today's difference is that rich browser-based client-side applications are now viable because of the changes we've seen over the past few years.
Resources on JS web applications:
Some good books:
- Alex MacCaw's book JavaScript Web Applications.
- Addy Osmani has several books, both hardcopy and online, see http://addyosmani.com/blog/ for a complete list.
- Mikito Takada's (a.k.a. Mixu) single page app online book: http://singlepageappbook.com
- MSDN has some great content on Javascript applications development, including the book-length description of their project Silk demo app
Here are some high-level resources (my JavaScript web applications primer part 2 has many more resources, including in-depth resources):
- Alex MacCaw's post "Asynchronous UIs - the future of web user interfaces"
- This Addy Osmani article has a section: "Why Might SPAs Can Be Better Than Multi-Page Applications?"
- Addy Osmani slide show with an overview of tech used in js web apps: Addy Osmani's slide deck for his talk "Tools for jQuery Application Architecture"
- Aaron Hardy's series of posts on JavaScript applications architecture
- http://msdn.microsoft.com/en-us/library/jj149689.aspx
- http://msdn.microsoft.com/en-us/library/jj149679.aspx
- Herding Code podcast: 155 – Ward Bell on Single Page Applications and Breeze
- JavaScript Jabber podcast: 041 JSJ Single Page Applications
- Tessa Harmon's Slideshare overview of SPAs
- Zdnet's recent Future of web apps article
And since there's a lot of talk these days about whether you should be creating mobile native apps or web apps (or hybrids) here are some interesting links on this topic:
- The Financial Times take on web apps v. native apps and Smashing has a case study (from 2013) on the FT web app
- Google IO video 2011 : Reto Meier and Michael Mahemoff "HTML5 v. Android: Apps or Web for Mobile"
- Michael Mahmedoff throws in the towel (2013): "Going native: Why a veteran web developer finally turned to OS-native apps"
- Paul Irish sends up distress flares (2013): "The mobile web is dying and needs your help"
- Nice overview of Native Apps, Web Apps, and Hybrid Apps
- An interesting slide deck looking at HTML5 web apps versus native apps for mobile (gets more interesting about midway...)
- An in-depth analysis of performance web apps v. native apps: "Why mobile web apps are slow" (comments here are useful, too)
- A view from Wired: "Native Apps vs. Mobile Web: Breaking Down the Mobile Ecosystem"
Related dlgsoftware posts
- Javascript web apps primer part 2
- JavaScript Data Explorer Overview (describes the SPA demo app)
- JavaScript Data Explorer (runs the SPA demo app)
Original version: October 2013
Copyright © Dan Gronell 2013. Licensed under the Creative Commons Attribution-Noncommercial-No Derivative Works 3.0 unported license.
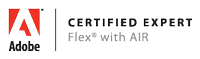
JavaScript
JavaScript appdev primers
General JavaScript
SPA Demo Application
Backbone Demo Application
Recommended sites
- Addy Osmani's blog
- Derick Bailey's Backbone posts
- Murphey's JQuery Fundamentals (original)
- Crockford's JS videos
- MSDN Project Silk
Flex/AIR
Flex/AIR primers
- Primer on Flex/AIR Multiscreen Development
- Primer on Mobile App Development w/Flex 4.5
- Primer on Flex 3 Component Lifecycle
- Primer on Flexlib MDI
Flex demo apps
all require Flash Player!
AIR mobile dev
- AIR mobile dev Tips
- AIR and Android Back key
- AIR, StageWebView, displaying local content
- AIR for Android memory issue w/large images